실습문제 1번
1. 일단 먼저 배열에 1~100까지의 숫자를 담는다.
2. 10개씩 출력해야하기 때문에 10개씩 끊어서 나눠서 개행문자를 넣어주어야만 한다.
10으로 나눴을때 0이 될 경우엔, 10번째 값을 가지고 있는 것이기에 if((i+1)%10==0)이라는 조건문을 넣어주었다.
그냥 i로만 나눌 경우엔 i가 0일 때도 포함되기에 원하는 결과값이 나오지 않는다.
<code>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
#include<iostream>
#include <string>
using namespace std;
int main(){
int arr[100] = { 0, };
for (int i = 0; i < 100; i++)
{
arr[i] = i+1;
cout << arr[i]<< '\t';
if ((i+1) % 10 == 0) cout << '\n';
}
}
|
cs |
<결과물>
실습문제 2번
구구단을 출력해야하기 때문에 맨 처음 숫자와 나머지 숫자를 구하기 위해선 이중 for문을 사용하면 된다..!
c와는 다르게 c++에서는 string line 문자열에 +=로 간단하게 문자열을 추가할 수 있다.
string line을 선언한 다음, int형 정수를 to_string 함수로 문자열로 변환한다음 합쳤다.
<code>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
#include <iostream>
#include<string>
using namespace std;
int main()
{
for (int i = 1; i <=9; i++)
{
string line = "";
for (int j = 1; j <= 9; j++) {
line += to_string(j);
line += 'x';
line += to_string(i);
line += '=';
line += to_string(j * i);
line += '\t';
}
cout << line << endl;
}
return 0;
}
|
cs |
<결과물>
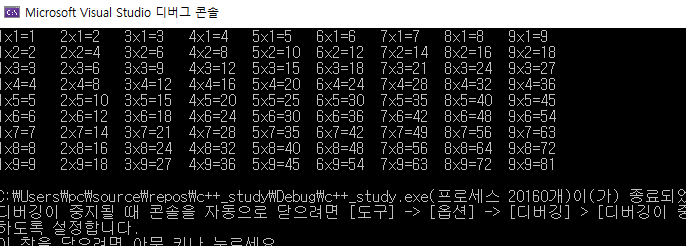
실습문제 3번
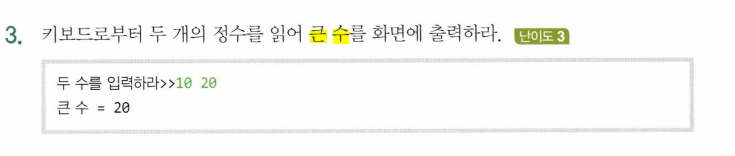
두 개의 큰 수를 출력하는 문제다. 정수 2개와 큰 값을 저장할 변수 하나를 더 세운 다음 출력해주면 된다.
<code>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
#include <iostream>
using namespace std;
int main()
{
int a, b, max = 0;
cout << "두 수를 입력하라>>";
cin >> a >> b;
if (a > b) max = a;
else max = b;
cout << "큰 수 =" << max << endl;
}
|
cs |
<출력화면>
실습문제 4번
위의 3번 문제랑 구성은 동일하다. 다만 숫자가 더 많아졌기에 배열에 담아서 숫자를 입력 받은 후 큰 값을 찾아 max에 저장한 후 출력한다.
<code>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
#include <iostream>
#include <algorithm>
using namespace std;
int main()
{
double a[5] = { 0, };
double max = 0;
cout << "5개의 실수를 입력하라>>";
for (int i = 0; i < 5; i++)
{
cin >> a[i];
}
for (int i = 1; i < 5; i++)
if (a[i] > max)max = a[i];
cout << "큰 수 =" << max << endl;
return 0;
}
|
cs |
<출력>
실습문제 5번
getline 함수를 사용하여 공백까지 함께 string을 입력을 받은 후, string 문자열 내에 있는 문자의 갯수를 세어주면 된다. 처음에는 헷갈리기 쉬운데 str.at() 함수를 쓰면 for문이 돌아가는 동안 문자열 내에 있는 배열의 인덱스 값을 검색할 수 있다. 해당 함수를 사용하면 쉽게 풀 수 있는 문제다.
<code>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
#include<iostream>
#include<string>
using namespace std;
int main() {
string str;
int cnt = 0;
cout << "문자들을 입력하라(100개 미만)." << endl;
getline(cin, str);
for (int i = 0; i < str.size(); i++)
{
if (str.at(i) == 'x')
cnt++;
}
cout << "x의 갯수는 " << cnt << endl;
}
|
cs |
<출력>
실습문제 6번
두 개의 문자열을 입력받고, 같은 지 비교하는 문제다. 간단하게 if문으로 비교문을 작성하면 된다.
<code>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
#include<iostream>
#include<string>
using namespace std;
int main() {
string code;
cout << "새 암호를 입력하세요>>";
cin >> code;
string n_code;
cout << "새 암호를 다시 한 번 입력하세요>>";
cin >> n_code;
if (code == n_code) cout << "같습니다";
else cout << "같지 않습니다";
}
|
cs |
<출력>
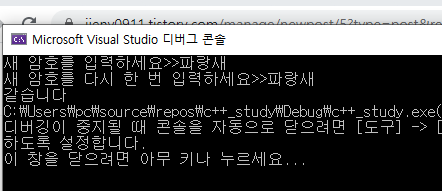
실습문제 7번
getline 함수를 써야지만 두번째 문장을 입력했을 때 종료되지 않는다.
종료되지 않게 하려면 while문을 사용하여 무한 반복을 하게 한 후, 원하는 조건문에 해당될때만 break가 걸리도록 설정해주면 된다.
<code>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
#include<iostream>
#include<string>
using namespace std;
int main() {
string yes;
while (1)
{
cout << "종료하고 싶으면 yes를 입력하세요>>";
getline(cin, yes);
if (yes == "yes") { cout << "종료합니다.."; break; }
}
}
|
cs |
<출력>
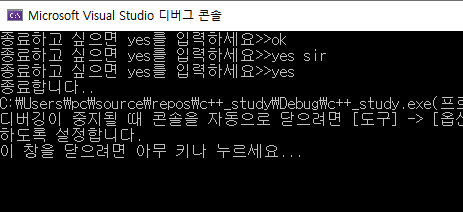
실습문제 8번
ㅠㅠ 위의 문제들보다 훨씬 어려운 문제 ㅠㅠ
많이 헤매고 다른 사람이 푼 코드를 보고 힌트를 얻어서 겨우 푼 문제.
cin.getline으로 name값을 받은 후에 그 중에 가장 긴 문자열을 찾는 문제다.
일단 for문으로 이름을 여러번 받은 다음, 그때마다 받은 문자열을 출력해준다.
그다음 문자열 길이를 비교하여 가장 긴 문자열을 찾는다.
len 이라는 변수를 두어서 가장 길게 찾은 문자열을 저장해두고 해당 문자열을 bigName으로 복사한다.
앞에 이름보다 더 긴이름이 나오면 bigName에 저장을 하는 것이다.
그 후 bigName을 출력해주면 된다.
<code>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
#include<iostream>
#include<string>
using namespace std;
int main()
{
cout << "5 명의 이름을 ';'으로 구분하여 입력하세요" << endl << ">>";
char name[100], bigName[100];
int len = 0;
for (int i = 0; i < 5; i++)
{
cin.getline(name, 100, ';');
cout << i + 1 << " : " << name << endl;
if (strlen(name) > len)
{
len = strlen(name);
strcpy_s(bigName, name);
}
}
cout << "가장 긴 이름은 " << bigName;
}
|
cs |
<출력>
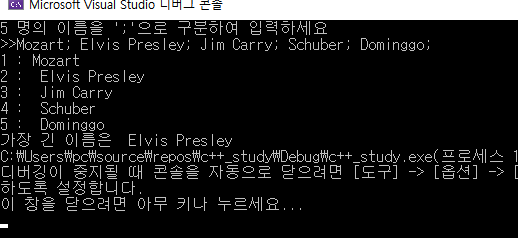
실습문제 9번
이 문제도 띄어쓰기가 들어가기 때문에 getline을 사용하면 된다.
<code>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
#include<iostream>
#include <string>
using namespace std;
int main()
{
string name, adrr, age, total;
cout << "이름은?";
getline(cin, name);
total += name;
total += ", ";
cout << "주소는?";
getline(cin, adrr);
total += adrr;
total += ", ";
cout << "나이는?";
getline(cin, age);
total += age;
total += "세";
cout << total;
}
|
cs |
<출력>
실습문제 10번
어떻게 해야하는 지 고민 많이 했는 데 생각보다 쉬웠다.
입력 받은 문자열은 하나하나씩 다른 문자열에 더해주면서 출력하는 방식으로 풀었다.
<code>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
#include<iostream>
#include <string>
using namespace std;
int main()
{
string str, tot;
cin >> str;
for (int i = 0; i < str.size(); i++)
{
tot += str.at(i);
cout << tot << endl;
}
return 0;
}
|
cs |
<출력>
실습문제 13번
어렵진 않으나 다소 귀찮은 문제...
while문 안에 switch 문을 사용해서 풀었다.
정수가 아닌 문자를 입력할 때 입력오류를 체크하기 위해서 확인하는 함수도 추가했다.
<code>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
|
#include<iostream>
#include <string>
using namespace std;
bool checkInputError() {
if (cin.fail()) { // 정수대신 문자열이 입력되어 오류가 발생하는 경우 대처. 부록 C의 예제 C-1참고
cin.clear();
cin.ignore(100, '\n');
cout << "입력 오류" << endl;
return true; // 오류 있음
}
else
return false; // 오류 없음
}
int main()
{
int num, num2;
cout << "***** 승리장에 오신 것을 환영합니다. *****"<<"\n";
while (1)
{
cout << "짬뽕:1, 짜장:2, 군만두:3, 종료:4>>";
cin >> num;
if (checkInputError()) continue;
switch (num)
{
case 1:
cout << "몇인분?";
cin >> num2;
if (checkInputError()) continue;
cout << "짬뽕 " << num2 << "인분 나왔습니다"<<endl;
break;
case 2:
cout << "몇인분?";
cin >> num2;
if (checkInputError()) continue;
cout << "짜장 " << num2 << "인분 나왔습니다" << endl;
break;
case 3:
cout << "몇인분?";
cin >> num2;
if (checkInputError()) continue;
cout << "군만두 " << num2 << "인분 나왔습니다" << endl;
break;
case 4:
cout << "오늘 영업은 끝났습니다. " << endl;
break;
default:
cout << "다시 주문하세요!" << endl;
break;
}
}
}
|
cs |
<출력>
실습문제 14번
strcmp를 사용해서 풀었다~! 20000원 매출을 벌면 종료되어야하기에 while문을 쓰더라도 조건문을 넣었다.
<code>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
|
#include<iostream>
#include <string>
using namespace std;
int main()
{
char coffee[100];
int num = 0;
int sale = 0;
int tot = 0;
cout << "에스프레소 2000원, 아메리카노 2300원, 카푸치노 2500원입니다."<<endl;
while (tot < 20000)
{
cout << "주문>>";
cin >> coffee >> num;
if (strcmp(coffee, "에스프레소") == 0)
{
sale = num * 2000;
tot += sale;
cout << sale << "원입니다. 맛있게 드세요" << endl;
}
if (strcmp(coffee, "아메리카노") == 0)
{
sale = num * 2300;
tot += sale;
cout << sale << "원입니다. 맛있게 드세요" << endl;
}
if (strcmp(coffee, "카푸치노") == 0)
{
sale = num * 2500;
tot += sale;
cout << sale << "원입니다. 맛있게 드세요" << endl;
}
if (tot > 20000)
cout << "오늘" << tot << "원을 판매하여 카페를 닫습니다. 내일 봐요~~" << endl;
}
return 0;
}
|
cs |
<출력>
실습문제 15번
공백이 있는 문자열을 입력 받은 후, strtok로 잘라서 연산을 하는 문제다.
ㅠ.ㅠ strtok는 맨날 해도 해도 어려워서 이 문제도 거의 한 시간 가까이 걸린 것 같다...
strtok로 자른 다음, 자른 값을 저장해줄 변수를 세워야함!
if문보다는 switch문을 좋아해서 이번에도 if문을 썼다.. if로 하면 일일히 조건문 작성해야해서 귀찮..
<code>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
|
#include<iostream>
#include <string>
using namespace std;
int main()
{
char cal[100];
char* ptr;
int front, rear;
while (1)
{
cout << "? ";
cin.getline(cal, 100);
front = atoi(strtok(cal, " "));
ptr = strtok(NULL, " ");
rear = atoi(strtok(NULL, " "));
switch (*ptr)
{
case '+':
cout << front << " " << ptr << " " << rear << " = " << front + rear << endl;
break;
case '-':
cout << front << " " << ptr << " " << rear << " = " << front - rear << endl;
break;
case '*':
cout << front << " " << ptr << " " << rear << " = " << front * rear << endl;
break;
case '/':
cout << front << " " << ptr << " " << rear << " = " << front / rear << endl;
break;
case '%':
cout << front << " " << ptr << " " << rear << " = " << front % rear << endl;
break;
default:
break;
}
}
}
|
cs |
<출력>
실습문제 16번
'C++ > 명품 c++ programming' 카테고리의 다른 글
명품 Programming C++ chapter3 챕터 3 실습 문제 5번 (2) | 2020.07.13 |
---|---|
명품 Programming C++ chapter3 챕터 3 실습 문제 4번 (0) | 2020.07.10 |
명품 Programming C++ chapter3 챕터 3 실습 문제 3번 (0) | 2020.07.10 |
명품 Programming C++ chapter3 챕터 3 실습 문제 1번 2번 (0) | 2020.07.10 |
명품 programming c++ open challenge 3장 (0) | 2020.07.10 |