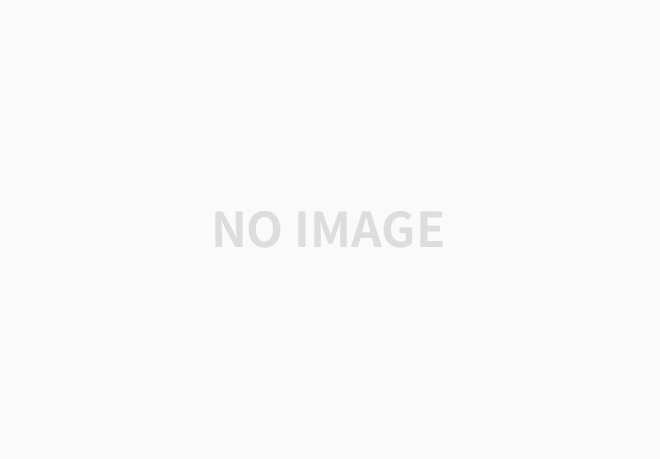
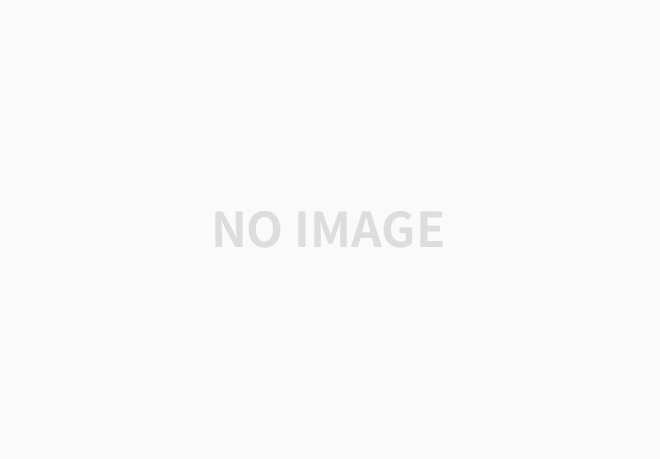
<code>
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include <iostream> | |
#include <string> | |
using namespace std; | |
class Circle | |
{ | |
int radius; | |
public: | |
Circle(int r) { radius = r; }; | |
int getRadius() { return radius; } | |
void setRadius(int r) { radius = r; } | |
void show() { cout << "반지름이 " << radius << "인 원" << endl; } | |
}; | |
void increaseBy(Circle& a, Circle& b) { | |
int r = a.getRadius() + b.getRadius(); | |
a.setRadius(r); | |
} | |
int main() | |
{ | |
Circle x(10), y(5); | |
increaseBy(x, y); | |
x.show(); | |
} |
'C++ > 명품 c++ programming' 카테고리의 다른 글
명품 Programming C++ chapter 5 챕터 5장 실습 문제 7번 (0) | 2020.08.03 |
---|---|
명품 Programming C++ chapter 5 챕터 5장 실습 문제 6번 (0) | 2020.08.03 |
명품 Programming C++ chapter 5 챕터 5장 실습 문제 4번 (0) | 2020.08.03 |
명품 Programming C++ chapter 5 챕터 5장 실습 문제 3번 (0) | 2020.08.03 |
명품 Programming C++ chapter 5 챕터 5장 실습 문제 2번 (0) | 2020.08.03 |